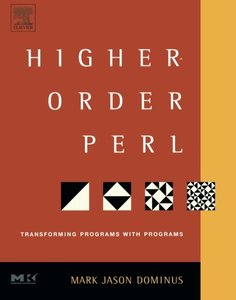
Higher-Order Perl: Transforming Programs with Programs
內容描述
Description:
"Higher-Order Perl is the most exciting, most clearly-written, most
comprehensive, and most forward-looking programming book I've read in at least
ten years. It's your map to the future of programming in any
language."—Sean M. Burke, Leading Programmer, Comprehensive Perl Archive
Network (CPAN) Most Perl programmers were originally trained as C and Unix
programmers, so the Perl programs that they write bear a strong resemblance to
C programs. However, Perl incorporates many features that have their roots in
other languages such as Lisp. These advanced features are not well understood
and are rarely used by most Perl programmers, but they are very powerful. They
can automate tasks in everyday programming that are difficult to solve in any
other way. One of the most powerful of these techniques is writing functions
that manufacture or modify other functions. For example, instead of writing
ten similar functions, a programmer can write a general pattern or framework
that can then create the functions as needed according to the pattern. For
several years Mark Jason Dominus has worked to apply functional programming
techniques to Perl. Now Mark brings these flexible programming methods that he
has successfully taught in numerous tutorials and training sessions to a wider
audience.
Table of
Contents:
- Recursion and Callbacks1.1 Decimal to Binary
Conversion1.2 Factorial1.2.1 Why Private Variables are
Important1.3 The Tower of Hanoi1.4 Hierarchical Data1.5
Applications and Variations of Directory Walking1.6 Functional vs.
Object-Oriented Programming1.7 HTML1.7.1 More Flexible
Selection1.8 When Recursion Blows Up1.8.1 Fibonacci Numbers1.8.2
Partitioning2. Dispatch Tables2.1 Configuration File
Handling2.1.1 Table-driven configuration2.1.2 Advantages of Dispatch
Tables2.1.3 Dispatch Table Strategies2.1.4 Default Actions2.2
Calculator2.2.1 HTML Processing Revisited3. Caching and
Memoization3.1 Caching Fixes Recursion3.2 Inline Caching3.2.1
Static Variables3.3 Good Ideas3.4 Memoization3.5 The Memoize
Module3.5.1 Scope and Duration3.5.1.1 Scope3.5.1.2
Duration3.5.2 Lexical Closure3.5.3 Memoization Again3.6
Caveats3.6.1 Functions whose Return Values do not Depend on their
Arguments3.6.2 Functions with Side Effects3.6.3 Functions that Return
References3.6.4 A Memoized Clock?3.4.5 Very Fast Functions3.7 Key
Generation3.7.1 More Applications of User-Supplied Key Generators3.7.2
Inlined Cache Manager with Argument Normalizer3.7.3 Functions with
Reference Arguments3.7.4 Partioning3.7.5 Custom Key Generation for
Impure Functions7.8 Caching in Object Methods3.8.1 Memoization of
Object Methods3.9 Persistent Caches3.10 Alternatives to
Memoization3.11 Evangelism3.12 The Benefits of Speed3.12.1
Profiling and Performance Analysis3.12.2 Automatic Profiling3.12.3
Hooks4. Iterators4.1 Introduction4.1.1 Filehandles are
Iterators4.1.2 Iterators are Objects4.1.3 Other Common Examples of
Iterators4.2 Homemade Iterators4.2.1 A Trivial Iterator: upto()4.2.1.1 Syntactic Sugar for
Manufacturing Iterators4.2.2 dir_walk()4.2.3 On Clever
Inspirations4.3 Examples4.3.1 Permutations4.3.2 Genomic Sequence
Generator4.3.3 Filehandle Iterators4.3.4 A Flat-File
Database4.3.4.1 Improved Database4.3.5 Searching Databases
Backwards4.3.5.1 A Query Package that Transforms Iterators4.3.5.2 An
Iterator that Reads Files Backwards4.3.5.3 Putting it Together4.3.6
Random Number Generation4.4 Filters and Transforms4.4.1 imap()4.4.2 igrep()4.4.3 list_iterator()4.4.4 append()4.5 The Semipredicate
Problem4.5.1 Avoiding the Problem4.5.2 Alternative undefs4.5.3 Rewriting Utilities4.5.4
Iterators that Return Mulitple Values4.5.5 Explicit Exhaustion
Function4.5.6 Four-Operation Iterators4.5.7 Iterator Methods4.6
Alternative Interfaces to Iterators4.6.1 Using foreach to Loop over more than one
Array4.6.2 An Iterator with an each-like Interface4.6.3 Tied Variable
Interfaces4.6.3.1 Summary of tie4.6.3.2 Tied Scalars4.6.3.3 Tied
Filehandle4.7 An Extended Example: Web Spiders4.7.1 Pursuing only
Interesting Links4.7.2 Referring URLs4.7.3 robots.txt4.7.4 Summary5. From
Recursion to Iterators5.1 The Partition Problem Revisited5.1.1
Finding All Possible Partions5.1.2 Optimizations5.1.3
Variations5.2 How to Convert a Recursive Function to an Iterator5.3 A
Generic Search Iterator5.4 Other General Techniques for Eliminating
Recursion5.4.1 Tail Call Elimination5.4.1.1 Someone Else's
Problem5.4.1.2 Creating Tail Calls5.4.1.3 Explicit Stacks5.4.1.3.1
Eliminating Recursion from fib()6. Infinite
Streams6.1 Linked Lists6.2 Lazy Linked Lists6.2.1 A Trivial
Stream: upto()6.2.2 Utilities for
Streams6.3 Recursive Streams6.3.1 Memoizing Streams6.4 The Hamming
Problem6.5 Regex String Generation6.5.1 Generating Strings in
Order6.5.2 Regex Matching6.5.3 Cutsorting6.5.3.1 Log Files6.6
The Newton-Raphson Method6.6.1 Approximation Streams6.6.2
Derivatives6.6.3 The Tortoise and the Hare6.6.4 Finance6.7 Power
Series6.7.1 Derivatives6.7.2 Other Functions6.7.3 Symbolic
Computation7. Higher-Order Functions and Currying7.1
Currying7.2 Common Higher-Order Functions7.2.1 Automatic
Currying7.2.2 Prototypes7.2.2.1 Prototype Problems7.2.3 More
Currying7.2.4 Yet More Currying7.3 reduce() and combine()7.3.1 Boolean Operators7.4
Databases7.4.1 Operator Overloading8. Parsing8.1
Lexers8.1.1 Emulating the <>
operator8.1.2 Lexers More Generally8.1.3 Chained Lexers8.1.4
Peeking8.2 Parsing in General8.2.1 Grammars8.2.2 Parsing
Grammars8.3 Recursive Descent Parsers8.3.1 Very Simple
Parsers8.3.2 Parser Operators8.3.3 Compound Operators8.4
Arithmetic Expressions8.4.1 A Calculator8.4.2 Left Recursion8.4.3
A Variation on star()8.4.4 Generic
Operator Parsers8.4.5 Debugging8.4.6 The Finished Calculator8.4.7
Error Diagnosis and Recovery8.4.7.1 Error Recovery Parsers8.4.7.2
Exceptions8.4.8 Big Numbers8.5 Parsing Regexes8.6 Outlines8.7
Databases Query Parsing8.7.1 The Lexer8.7.2 The Parser8.8
Backtracking Parsers8.8.1 Continuations8.8.2 Parse Streams8.9
Overloading9. Declarative Programming9.1 Constraint
Systems9.2 Local Propagation Networks9.2.1 Implementing a Local
Propagation Network9.2.2 Problems with Local Propagation9.3 Linear
Equations9.4 linogram: a drawing
system9.4.1 Equations9.4.1.1 ref($base) || $base9.4.1.2 Solving
Equations9.4.1.3 Constraints9.4.2 Values9.4.2.1 Constant
Values9.4.2.2 Tuple Values9.4.2.3 Feature Values9.4.2.4 Intrinsic
Constraints9.4.2.5 Synthetic Constraints9.4.2.6 Feature Value
Methods9.4.3 Feature Types9.4.3.1 Scalar Types9.4.3.2 Type methods9.4.4 The Parser9.4.4.1
Parser Extensions9.4.4.2 %TYPES9.4.4.3 Programs9.4.4.4
Definitions9.4.4.5 Declarations9.4.4.6 Expressions9.4.5 Missing
Features9.5 Conclusion